Rust Developer Bootcamp
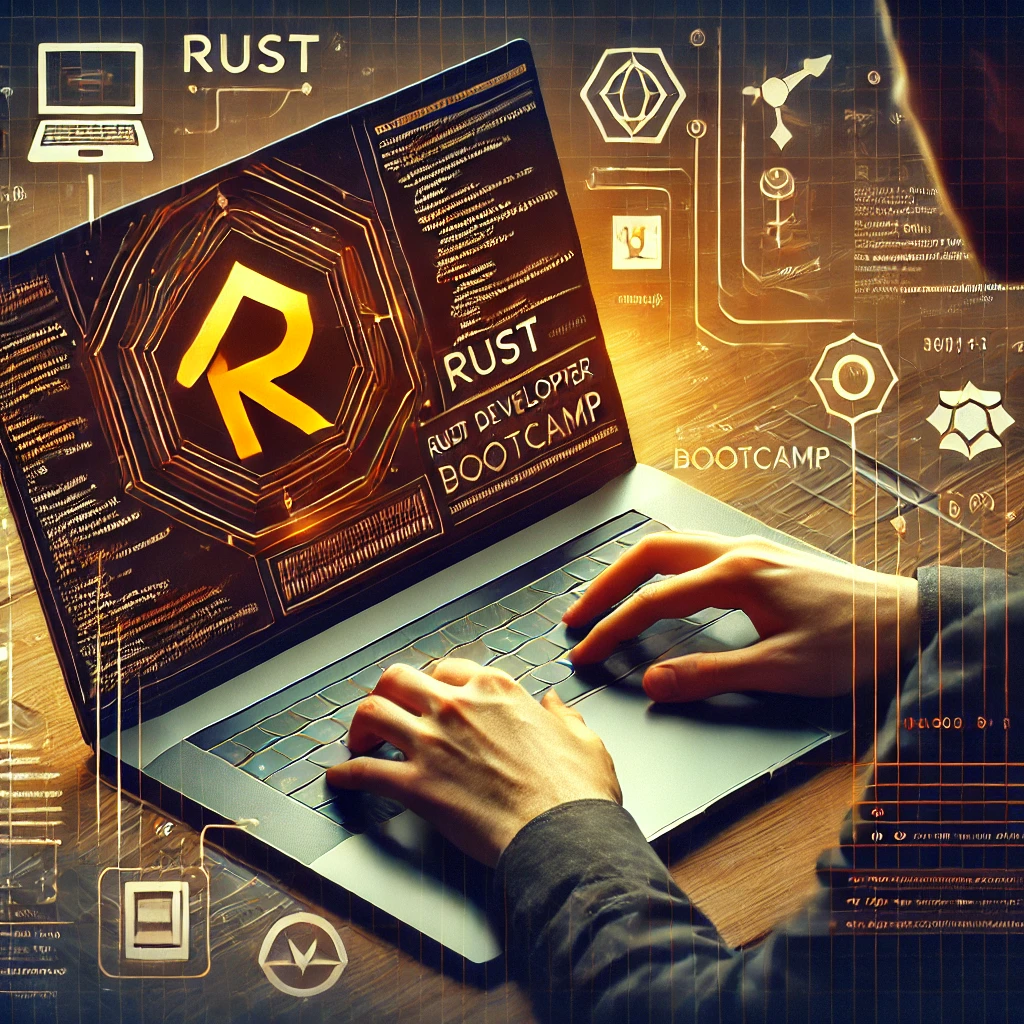
About Course
Looking to make the leap to Rust or enhance your programming repertoire? This bootcamp is tailored for experienced developers ready to transition to Rust, students aiming to stand out in the job market, and passionate Rust enthusiasts eager to explore a cutting-edge language. Gain a comprehensive understanding of Rust, one of the most innovative programming languages, known for its safety, performance, and versatility.
Who Should Join This Bootcamp?
Experienced Developers: Transition smoothly to Rust and learn the language faster without wasting time on scattered, low-quality resources. Boost your earning potential to around $148,688/year by mastering this high-demand skill.
Students & Graduates: Stand out in the competitive job market with Rust on your resume. Develop your programming competence and connect with a supportive community to help you land your first job.
Rust Enthusiasts: Deepen your knowledge of Rust, expand your thinking, and explore how this language powers some of today’s most innovative technologies.
Course Highlights: A Step-by-Step Journey into Rust You’ll begin by setting up your development environment, including configuring your IDE and necessary plugins, before diving into your first Rust project. Here’s what you can expect to learn:
Setting Up Your Development Environment
Customizing your IDE and plugins
Creating your first Rust project using Cargo
Understanding variables, constants, and functions
Grasping fundamental concepts of memory management
Mastering Memory Management
A foundational aspect of Rust, memory management is taught from the ground up:
Ownership and borrowing mechanisms
Understanding slices and string types (str, &str, String)
Creating Custom Data Types
Learn how Rust handles data structures differently from other languages:
Defining and using structs and enums
Working with implementation blocks and pattern matching
Handling data using Option and Result enumerations
Building and Structuring Rust Projects
Organize your code efficiently and share your work:
Project structure overview and the module system
Managing dependencies and conditional compilation
Publishing your projects on Crates.io
Writing Reliable Code with Testing & Documentation
Ensure your code is robust and well-documented:
Creating unit and integration tests
Best practices for test structure and documentation
Achieving Polymorphism with Generics and Traits
Rust’s alternative to classical inheritance:
Defining generics and understanding traits
Navigating trait boundaries, supertraits, and dispatching
Using traits from Rust’s standard library
Advanced Memory Management & Lifetimes
Deepen your understanding of Rust’s memory safety:
Concrete and generic lifetime annotations
Working with smart pointers and deref coercion
Error Handling in Rust
Develop resilient applications by mastering Rust’s approach to errors:
Generating and handling errors
Using Result and Option for error propagation
Employing libraries like anyhow and thiserror for effective error handling
Leveraging Functional Features in Rust
Harness Rust’s powerful functional programming capabilities:
Using closures, function pointers, and iterators
Applying combinators for more efficient code
Parallelism & Async Programming
Understand parallelism to build scalable, high-performance applications:
Basics of threading, messaging, and state sharing with Mutex
Mastering async programming with async/.await and understanding futures
Utilizing Tokio for asynchronous execution and task management
Expanding Your Skills with Rust’s Macro System
Explore Rust’s macro system for extending language syntax:
Creating declarative and procedural macros
Understanding function-like, attribute-like, and custom derive macros
Unlocking Rust’s Interoperability with Unsafe Code & FFI
Discover how Rust can interface with other languages, enhancing its versatility:
Using unsafe Rust and when it’s appropriate
Interacting with raw pointers and modifying static variables
Writing inline assembly and calling C code from Rust (and vice versa)
Why Choose This Bootcamp? This bootcamp offers a structured, hands-on approach to learning Rust, focusing on practical applications and real-world scenarios. By the end of the course, you’ll not only understand Rust’s unique features but also be equipped to apply them confidently in your projects. Expand your skill set, improve your programming efficiency, and prepare to thrive in a future where Rust continues to grow in demand.
Course Content
All lessons
-
001. Don’t skip this video!
-
002. How to use this Bootcamp effectively
-
003. Hello World
-
004. Variables
-
005. Data Types
-
006. Constants & Statics
-
007. Functions
-
008. Control Flow
-
009. Comments
-
010. Stack, Heap, and Static Memory
-
011. Memory Management Strategies
-
012. C++ RAII vs Rust OBRM – Part 1
-
013. C++ RAII vs Rust OBRM – Part 2
-
014. Ownership
-
015. Ownership Continued
-
016. Borrowing
-
017. Slices
-
018. BONUS Masterclass Strings in Rust. PART 1
-
019. BONUS Masterclass Strings in Rust. PART 2
-
020. Structs
-
021. Implementation Blocks
-
022. Tuple Structs
-
023. Enums
-
024. Matching
-
025. Option
-
026. Result
-
027. Vectors
-
028. Project Structure Overview
-
029. Modules
-
030. Modules Continued
-
031. External Dependencies
-
032. Publishing Your Package
-
033. Cargo Features
-
034. Cargo Workspaces
-
035. Unit Tests
-
036. Integration Tests
-
037. Documentation
-
038. BONUS Benchmark Testing
-
039. Generics
-
040. Traits
-
041. Trait Bounds
-
042. Supertraits
-
043. Trait Objects
-
044. Deriving Traits
-
045. The Orphan Rule
-
046. Concrete Lifetimes
-
047. Generic Lifetimes
-
048. Structs Lifetime Elision
-
049. Box Smart Pointer
-
050. Rc Smart Pointer
-
051. RefCell Smart Pointer
-
052. Deref Coercion
-
053. Unrecoverable Errors
-
054. Recoverable Errors
-
055. Propagating Errors
-
056. Result and Option
-
057. Multiple Error Types
-
058. Overview of Error Handling
-
059. Basic Error Handling
-
060. Custom Errors 1
-
061. Custom Errors 2
-
062. Custom Errors 3
-
063. thiserror & anyhow
-
064._BONUS_error-stack
-
065. Closures
-
066. Closures Continued
-
067. Function Pointers
-
068. Iterator Pattern
-
069. Iterator Pattern Continued
-
070. Iterating Over Collections
-
071. Combinators
-
072. Intro to Concurrency
-
073. Creating Threads
-
074. Moving Values Into Threads
-
075. Message Passing Between Threads
-
076. Sharing State Between Threads
-
077. Sharing State Between Threads Continued
-
078. Send & Sync Traits
-
079. async.await Basics
-
080. Tokio Tasks
-
081. CPU Intensive Code
-
082. Streams
-
083. Intro to Macros
-
084. Declarative Macros
-
085. Declarative Macros Continued
-
086. Procedural Macros
-
087. Procedural Macros – Function Like
-
088. Procedural Macros – Custom Derive
-
089. Procedural Macros – Attribute Like
-
090. Procedural Macros – Attribute Continued
-
091. Unsafe Basics
-
092. Dereferencing a Raw Pointer
-
093. Calling an Unsafe Function
-
094. Implementing an Unsafe Trait
-
095. Mutable Static Variables
-
096. Inline Assembly
-
097. FFI C from Rust
-
098. FFI Rust from C
-
099. BONUS FFI Rust from Python
-
100. What are microservices
-
101. Why Rust is great for microservices
-
102. Communication with gRPC
-
103. Containerization with Docker
-
104. CICD with GitHub Actions
-
105. Cloud Providers